When taking samples or deciding which places to record observations, for example along a transect line, we need sometimes to choose sites randomly. Imagine a transect line that’s 50 meters long, and we want to take samples at random intervals, either left or right and between one and three meters of the transect line. A very efficient way to do this is using a short python script.
The following python code creates three lists, one for the direction of offset left and right, one list with the distance of offset, and one choosing a random distance along the transect. These lists are then stacked and sorted, giving in this example 10 random samples along a 50m transect.
import numpy as np
import random
offset_direction = [“Left”, “Right”]
offset_distance =[“1m”, “2m”, “3m”]
transectpoint=random.sample(range(1,50),10);
sorted_tps=np.sort(transectpoint, axis = 0)
offset_dir = np.random.choice(offset_direction,10, replace=True)
offset_dis = np.random.choice(offset_distance,10, replace=True)
random_lists = np.column_stack((sorted_tps, offset_dir, offset_dis))
print (random_lists)
which returns:
[[‘3’, ‘Left’, ‘2m’],
[‘4’, ‘Left’, ‘3m’],
[‘7’, ‘Left’, ‘3m’],
[’10’, ‘Right’, ‘1m’],
[’13’, ‘Left’, ‘1m’],
[’14’, ‘Left’, ‘2m’],
[’21’, ‘Right’, ‘3m’],
[’27’, ‘Right’, ‘3m’],
[’34’, ‘Left’, ‘1m’],
[’40’, ‘Left’, ‘3m’]]
You can copy and modify this code to suit your needs, paste it into any python terminal and get random!
Below the code is annotated to explain what’s going on.
first we set up the libraries we need, numpy and random
import numpy as np
import random
create the lists, these can be numbers or labels
offset_direction = [“Left”, “Right”]
offset_distance =[“1m”, “2m”, “3m”]
now we select the range of our transect line (50m) and how many samples we want (10)
change these numbers based on your needs
transectpoint=random.sample(range(1,50),10);
the transectpoint list is sorted from near to far
sorted_tps=np.sort(transectpoint, axis = 0)
two more sets of 10 random values are generated
one for the offset direction Left or Right
one for the offset distance 1m to 3m
replace=True allows us to have repeat values
offset_dir = np.random.choice(offset_direction,10, replace=True)
offset_dis = np.random.choice(offset_distance,10, replace=True)
then we stack up the random list
random_lists = np.column_stack((sorted_tps, offset_dir, offset_dis))
finally we print the new random values set
print (random_lists)
which outputs each time a new list
[[’12’ ‘Left’ ‘1m’]
[’15’ ‘Left’ ‘2m’]
[’21’ ‘Right’ ‘1m’]
[’22’ ‘Left’ ‘2m’]
[’24’ ‘Left’ ‘2m’]
[’27’ ‘Left’ ‘3m’]
[’32’ ‘Right’ ‘2m’]
[’34’ ‘Right’ ‘3m’]
[’35’ ‘Right’ ‘1m’]
[’43’ ‘Right’ ‘2m’]]
If you were to do this often, to make it easy the code can be made into an executable file. Here’s how to (on a Mac)
Add the following as the first line of code
#!/usr/bin/env python
save the file as random_lists.command
In a Terminal window, navigate to the folder containing the file and type
chmod +x random_lists.command
Now when you double-click this file it should open in a terminal window with your new list
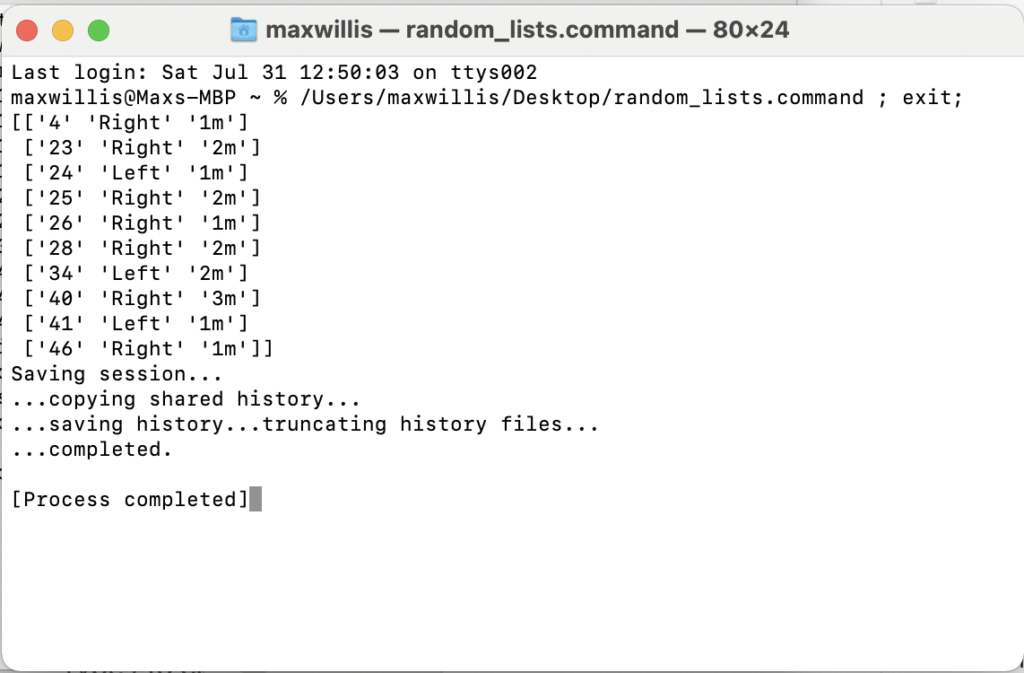